About the PHP Course
PHP stands for Hypertext Preprocessor. It is a free, open-source scripting language used to build websites. It works well with other technologies like HTML, CSS, JavaScript, and can run on any server or platform.
PHP code runs on the server and is turned into plain HTML that shows on your browser. It can be used to:
✅ Create website content
✅ Send and receive cookies
✅ Read, write, save, or delete files
✅ Encrypt data for security
PHP supports many types of databases and is easy to learn — from writing simple lines of code to building full websites. Since websites often need changes and updates, PHP developers are always in demand.
What You’ll Get
✅ Job Support
✅ Easy-to-follow Learning Materials
✅ Training by Experienced Professors
✅ Real-World Projects to Practice

Course Duration
12 Months

Daily Learning Time
2 Hours
PHP Development Training Course Objectives
🔹Learn how websites are created and how they work.
🔹Understand how server-side programming works using PHP.
🔹Write basic PHP code using variables and simple calculations.
🔹Use if-else and other conditional statements in PHP.
🔹Save and manage data using arrays.
🔹Use PHP’s built-in functions and create your own functions.
🔹Learn how POST and GET work in form submissions.
🔹Collect and process form data using PHP.
🔹Work with sessions to store user information.
🔹Use HTML designs and connect them with PHP code.
🔹Create and manage a company-related database using MySQL.
🔹Learn how to add security features to a website.
🔹Handle files (upload, read, write, etc.) in PHP.
🔹Understand and manage the website’s admin/backend panel.
🔹Learn Object-Oriented Programming (OOP) with PHP.
🔹Use AJAX for faster, smoother web experiences.
🔹Add social media features to websites.
🔹Send emails using PHP.
🔹Upload files through websites using PHP.
🔹Convert website data into an API format.
🔹Create a working payment gateway system.
🔹Build a complete blog or e-commerce website as a real project.
Core PHP
🔹Introduction to PHP
• What is PHP and how it's used
• Introduction to servers and installing XAMPP
• Using echo and print to display output
🔹Basics of PHP
• Data types, variables, and constants
• Using operators (arithmetic, assignment, logical)
• Writing comments in code
• Understanding $_REQUEST['get'] for form handling
🔹Control Structures
• Using if, if-else, else-if ladder, and nested if
• Using switch case and ternary operator
🔹Loops in PHP
• while, do-while, for, and nested loops
🔹Arrays and Functions
• Types of arrays: Indexed, Associative, Multidimensional
• Using foreach loop
• Built-in array, string, date, and time functions
• Creating your own functions (User Defined Functions)
🔹File Handling
• Basics of file systems
• Reading, writing, and handling files
• Using file and directory functions
🔹Working with Databases (MySQL)
• Introduction to databases and phpMyAdmin
• Creating databases and tables
• Basic CRUD operations: Insert, View, Update, Delete
• Importing/exporting SQL files
🔹PHP with Forms and CRUD
• How client-server communication works (HTTP methods)
• Connecting PHP with HTML forms
• Using super global variables
• CRUD with PHP:
• Insert data
• View data
• Update data
• Delete data
🔹Object-Oriented Programming (OOP)
• Introduction to OOP concepts
• Creating classes and methods
• CRUD operations using OOP:
• Insert, view, update, delete data
🔹Admin Panel in PHP
• Converting an HTML admin panel to PHP
• Using include(), require(), etc.
• Creating forms and tables
• Managing blog data:
• Add categories and subcategories
• Create blog posts
• Upload and manage multiple images
🔹Frontend Integration
• Convert HTML into dynamic PHP pages
• Add next/previous buttons with sliders
• Add comment section
• Add server-side validation
• Use regex to find patterns in data
🔹User Sessions
• Create login and logout functionality
• Handle multiple user logins
• View and update user profiles
• Change passwords
🔹JavaScript & jQuery (Frontend Validation)
• Introduction to JavaScript
• Data types, functions, and events
• Quantity increment/decrement feature
• Validate forms using JavaScript and jQuery
🔹AJAX
• Introduction to AJAX
• Using AJAX with database for:
• Insert data
• View/delete data
• Update data
• Live search
🔹Mailing in PHP
• Sending emails from a PHP website
• "Forgot password" email feature
LARAVEL
🔹Getting Started with PHP
• What is PHP and where is it used?
• Introduction to servers and installing XAMPP
• Displaying output using echo and print
🔹PHP Basics
• Data types in PHP
• Using variables and constants
• Introduction to operators (arithmetic, assignment, logical)
• Writing comments in PHP
• Using $_REQUEST['get'] to handle form data
🔹Conditional Statements
• if, if-else, else-if ladder, nested if-else
• switch case and ternary (? :) operator
🔹Loops in PHP
• while, do-while, for, and nested for loops
🔹Arrays and Functions
• Types of arrays:
• Indexed Arrays
• Associative Arrays
• Multidimensional Arrays
• foreach loop
• Useful array functions
• String and date/time functions
• Creating your own functions (User-Defined Functions)
🔹Working with Databases (SQL)
• Introduction to databases and phpMyAdmin
• Creating databases and tables
• Understanding primary and foreign keys
🔹Laravel Basics & Blade Template
• Intro to Laravel’s Blade templating engine
• Template inheritance
• Components and slots
• Showing data in views
• Using control structures in Blade
• Creating forms with Blade
🔹Admin Panel with Laravel
• Converting an HTML admin panel into Laravel
• Setting up a dashboard
• Creating tables and forms with MVC pattern
• CRUD operations using Laravel:
• Insert, View, Update, Delete
• Handling passwords with sha()
• CSRF protection in forms
🔹File Handling in Laravel
• Uploading a single file (Insert, View, Update, Delete)
• Uploading multiple files (Insert, View, Delete)
🔹Advanced Form Features
• Searching data using form fields
• Pagination (splitting results across pages)
• Combining search with pagination
• Form validation using Laravel's request system
🔹Blog Module
• Creating blog posts with multiple image uploads
• Viewing, deleting, and updating multiple images
🔹Client-Side Features
• Converting static HTML pages to dynamic PHP
• Making data dynamic
• Next/Previous buttons with sliders
• Adding comment functionality
🔹User Management
• Understanding sessions
• Simple login/logout system
• Multiple user login
• View, update profile, and change password
🔹JavaScript & jQuery (Frontend)
• Intro to JavaScript and its data types
• Writing functions and handling events
• Quantity increase/decrease (like in shopping carts)
• Validating forms with JavaScript
• jQuery form validation
🔹AJAX (Asynchronous Requests)
• What is AJAX and why it's used
• Using AJAX with databases:
• Insert, View, Update, Delete
• Live search functionality
🔹Emailing Features
• Sending emails using PHP or Laravel
• "Forgot Password" email functionality
CODEIGNITER
🔹Introduction to CodeIgniter
• What is MVC (Model-View-Controller)?
• Key features of MVC
• Why CodeIgniter is important and useful
🔹Installing CodeIgniter
• How to download and set up CodeIgniter
• Configuring the .env file
• Using authentication facades (login features)
🔹Understanding CodeIgniter Structure
• Folder structure and how everything is organized
• How URLs work in CodeIgniter
• How to remove index.php from the URL
🔹Controllers & Models
• What is a controller and how to create one
• How to call a controller and use constructors
• What is a model and how to load it in your code
🔹Routing
• Creating custom URL routes
• Using wildcards (dynamic values in URL)
• Redirecting to other pages
🔹Views
• Creating view files (HTML pages)
• Sending data to views from the controller
🔹Request & Response Handling
• Working with session data
• Flash data (temporary messages)
• Page redirects
• Managing cookies
🔹Database & SQL
• Introduction to databases and phpMyAdmin
• Creating databases and tables
• Understanding primary key and foreign key
🔹Admin Panel with CodeIgniter
• Converting an HTML admin panel into CodeIgniter
• Setting up the dashboard, tables, and forms
🔹File System
• Uploading a single file
• Uploading multiple files
🔹Form Features
• Search using form fields
• Add pagination (break content into pages)
• Combine search with pagination
• Validate form data before submission
🔹Working with Data
• Using JOIN queries to combine tables
• Fetch data using AJAX (without page reload)
🔹Admin Security
• Admin login and logout
• View and update profile
• Change password
🔹E-Commerce Website (Client Side)
• Set up the client side of the website in CodeIgniter
• Display all products
• Show single product details
• Filter products by category, price, etc.
🔹Client Security
• Client login and logout
• View and update profile
• Change password
🔹Shopping Cart
• Add products to cart
• View cart items
• Update or remove items from the cart
🔹Final Project
• Add mailing features (send emails)
• Set up payment gateway for online payments
PHP Training Course Overview
Why Choose PHP as a Career?
PHP is an easy-to-learn programming language that is widely used by developers. It's simple, works well on all systems, and is open-source, meaning anyone can use and improve it. PHP is flexible, allowing developers to make changes and updates easily. It's the language behind many popular websites, making it a highly versatile option for developers. With PHP, you can customize websites to meet specific needs, and you can add plugins and extensions to keep things updated. Given its flexibility, PHP remains one of the most favored programming languages for web development. If you're looking to start a career in web development, we are the leading provider of Web Development Courses in Mumbai, and we're here to help you get started!
Scope of PHP Developer
PHP is in high demand, both in India and globally, as many companies are adopting it for their web development needs. With strong knowledge of PHP, developers can find plenty of job opportunities. The field offers great earning potential, especially for those with multiple certifications in IT development, as it’s one of the most cost-effective ways to build a career in technology. PHP developers have various roles to choose from, such as web developers (designing and managing websites), or database managers (manipulating data).
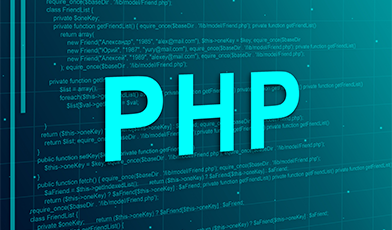